/*===C-Scan Scheduling===*/
#include<stdio.h>
#include<conio.h>
#include<graphics.h>
void denotion();
void main ()
{
int gd=DETECT,gm,m,a[30],i,n,j=100,o;
clrscr();
printf("==Circular-SCAN Disk Scheduling==\n\n");
printf("Total no of cylinders in the disk : 0 to 100\n");
printf("Enter the total no. of pending requests : ");
scanf("%d",&m);
printf("Enter the pending values : ");
for(i=1;i<=m;i++)
{
scanf("%d",&a[i]);
}
printf("Enter the value where the head initially exists : ");
scanf("%d",&n);
a[0]=n;
for(i=0;i<m;i++)
{
for(j=i+1;j<=m;j++)
{
if(a[j]<a[i])
{
o=a[i];
a[i]=a[j];
a[j]=o;
}
}
}
initgraph(&gd,&gm,"C://TC//BGI");
denotion();
for(i=0;i<=500;i+=5)
{
setcolor(WHITE);
rectangle(i,85,5+i,95);
if(i==0)
{
outtextxy(i,70,"0");
}
if(i==50)
{
outtextxy(i,70,"10");
}
if(i==100)
{
outtextxy(i,70,"20");
}
if(i==150)
{
outtextxy(i,70,"30");
}
if(i==200)
{
outtextxy(i,70,"40");
}
if(i==250)
{
outtextxy(i,70,"50");
}
if(i==300)
{
outtextxy(i,70,"60");
}
if(i==350)
{
outtextxy(i,70,"70");
}
if(i==400)
{
outtextxy(i,70,"80");
}
if(i==450)
{
outtextxy(i,70,"90");
}
if(i==500)
{
outtextxy(i,70,"100");
}
}
j=100;
for(i=0;i<m;i++)
{
if(a[i]==n)
{
setcolor(WHITE);
line(a[i]*5,j,a[i+1]*5,20+j);
setcolor(8);
setfillstyle(1,8);
circle(a[i+1]*5,20+j,5);
floodfill(a[i+1]*5,20+j,8);
delay(1);
j+=20;
setcolor(YELLOW);
setfillstyle(1,YELLOW);
rectangle(a[i]*5,85,(a[i]*5)+5,95);
floodfill((a[i]*5)+3,90,YELLOW);
delay(10);
}
if(a[i]>n)
{
setcolor(WHITE);
line(a[i]*5,j,a[i+1]*5,20+j);
setcolor(8);
setfillstyle(1,8);
circle(a[i+1]*5,20+j,5);
floodfill(a[i+1]*5,20+j,8);
delay(1);
j+=20;
setcolor(RED);
setfillstyle(1,RED);
rectangle(a[i]*5,85,(a[i]*5)+5,95);
floodfill((a[i]*5)+3,90,RED);
delay(10);
}
}
setcolor(RED);
setfillstyle(1,RED);
rectangle(a[m]*5,85,(a[m]*5)+5,95);
floodfill((a[m]*5)+3,90,RED);
delay(10);
if(a[0]!=n)
{
setcolor(WHITE);
line(a[m]*5,j,500,j+20);
line(500,j+20,0,j+50);
line(0,j+50,a[0]*5,j+70);
setcolor(8);
setfillstyle(1,8);
circle(a[0]*5,j+70,5);
floodfill(a[0]*5,j+70,8);
delay(10);
j+=70;
for(i=0;a[i+1]<n;i++)
{
setcolor(WHITE);
line(a[i]*5,j,a[i+1]*5,20+j);
setcolor(8);
setfillstyle(1,8);
circle(a[i+1]*5,20+j,5);
floodfill(a[i+1]*5,20+j,8);
delay(1);
j+=20;
setcolor(RED);
setfillstyle(1,RED);
rectangle(a[i]*5,85,(a[i]*5)+5,95);
floodfill((a[i]*5)+3,90,RED);
delay(10);
}
setcolor(RED);
setfillstyle(1,RED);
rectangle(a[i]*5,85,(a[i]*5)+5,95);
floodfill((a[i]*5)+3,90,RED);
delay(10);
}
getch();
closegraph();
}
void denotion()
{
outtextxy(200,20,"== C-Scan Disk Scheduling ==");
setcolor(YELLOW);
setfillstyle(1,YELLOW);
rectangle(520,380,525,390);
floodfill(523,385,YELLOW);
outtextxy(530,383,"-> Initial");
outtextxy(544,395,"position");
delay(1);
setcolor(RED);
setfillstyle(1,RED);
rectangle(520,420,525,430);
floodfill(523,425,RED);
outtextxy(530,423,"-> Request");
outtextxy(536,435,"cylinders");
delay(1);
}
Output :
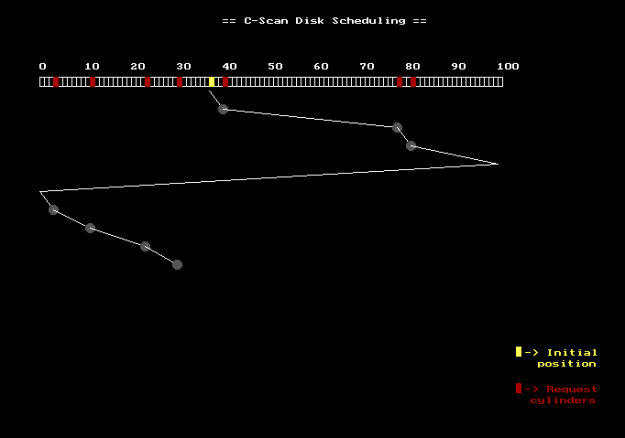
That’s All. P)
Happy C-ing!
-Aayush Shrivastava